Core Concepts
Modo is a state-based navigation library for Jetpack Compose. It represents the UI as a structure of Screen
s and ContainerScreen
s (which are implementations of Screen
).
Navigation is a Graph
Each integration of Modo is a rooted tree (wiki) that can be displayed as follows:
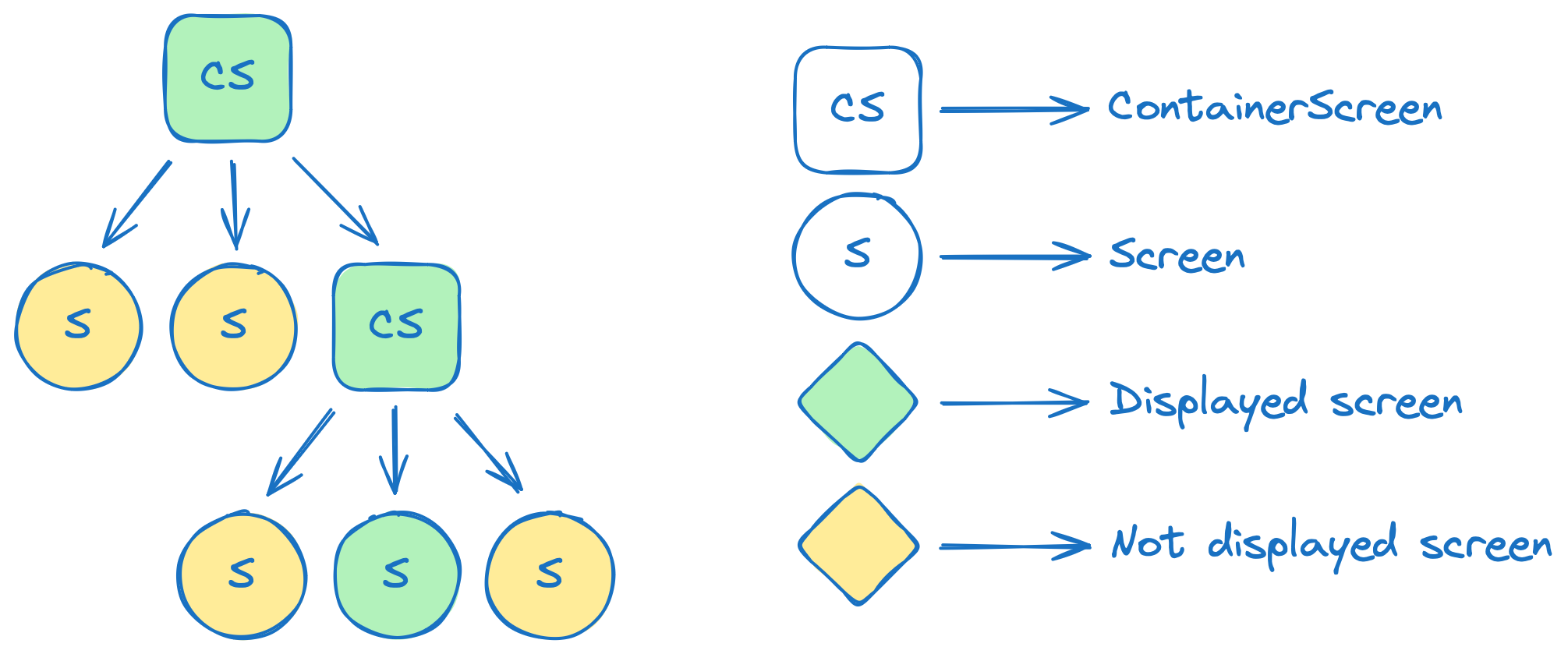
Each node is a
Screen
orContainerScreen
.Leaf nodes are
Screen
s.Inner nodes are
ContainerScreen
s. They can contain otherScreen
s orContainerScreen
s in theirnavigationState
.The root node is a
RootScreen
. You can have multiple roots in your app. See How to integrate Modo for details.
Screen
A Screen
is the basic unit of the UI. It displays content defined in the overridden fun Content(modifier: Modifier)
.
Screen Key
Each screen is identified by a ScreenKey
- a unique key representing the screen. This key is extensively used in internal implementation. It must be unique for each screen, even after process death. To ensure this, use the built-in generateScreenKey
function.
Arguments
To pass arguments to the screen, declare them in the Screen's constructor:
Saving and Restoring
Each screen is Parcelable
, which helps to save and restore it during lifecycle changes. Use the parcelable Gradle plugin and the @Parcelize
annotation to generate the Parcelable
implementation on the fly.
It's crucial to use built-in functions like rememberRootScreen
to integrate Modo with your application. Read How to Integrate Modo into Your App for details.
ContainerScreen
ContainerScreen
s are types of screens that can contain other screens. They are fundamental building blocks for complex navigation structures. StackScreen
and MultiScreen
are built-in implementations of ContainerScreen
.
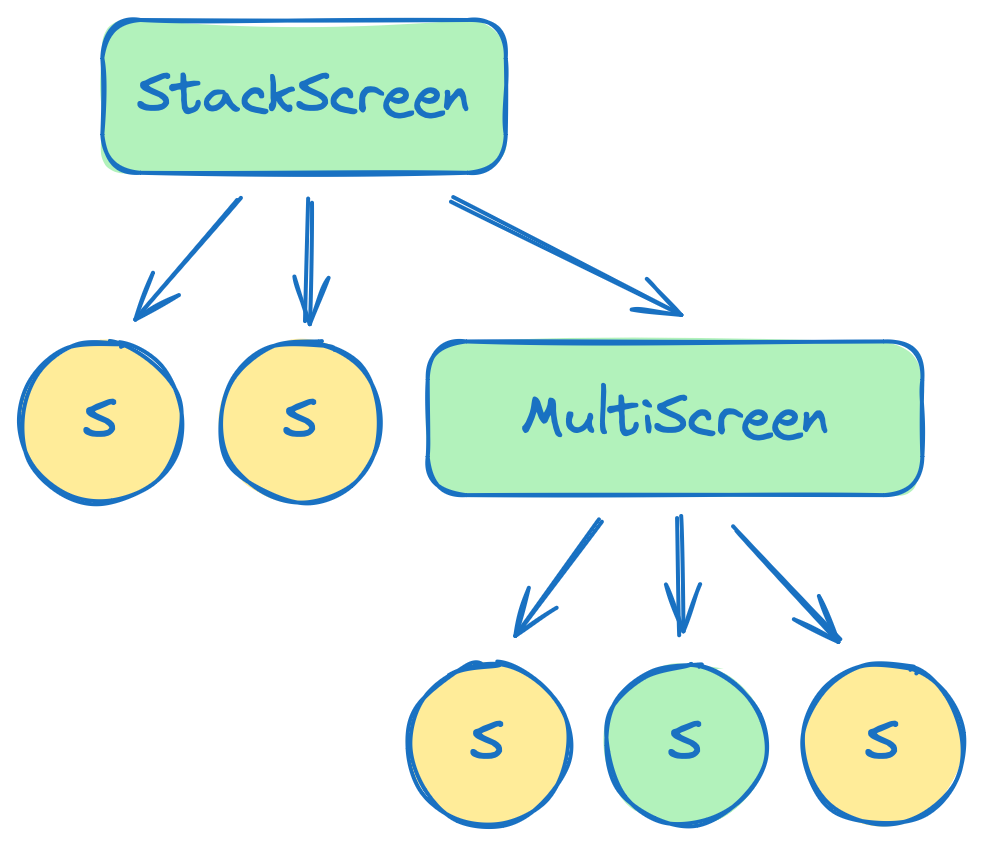
Each ContainerScreen is defined by two typed parameters: State and Action.
State
NavigationState
- a class that can contain nested screens and other additional information. The state can be updated by calling dispatch(action)
.
Read the State Update section for more details.
Action
NavigationAction
- a marker interface to distinguish actions for this container on a specific State
. You can also use ReducerAction
to define actions with an in-place update function:
NavModel
- a state storage responsible for state updates and triggering UI updates. Each ContainerScreen has a NavModel as a constructor parameter.
Rendering Nested Screens
To render nested screens inside a container screen, you must use the InternalContent
function. This function provides all necessary integrations, such as:
Correct usage of
rememberSaveable
inside nested screens by usingSaveableStateHolder
.Integration of
ScreenModel
, ensuring consistency for the same screen and clearing it when theScreen
leaves the hierarchy.Android integration, including
Lifecycle
andViewModel
support.
The built-in StackScreen
and MultiScreen
use InternalContent
under the hood to ensure correct nested screen functionality.
State Update
To update the state of a ContainerScreen
, use dispatch(action: Action)
. There are two ways to define your action:
ReducerAction (Recommended)
ReducerAction allows defining the update function in-place.
Custom Reducer + Action
You can provide a reducer in your ContainerScreen
implementation.
Root Screen
To integrate Modo into your application, use one of the built-in functions from the Modo file. It returns a RootScreen
, which provides a SaveableStateHolder
.